For and forEach are both ways to loop over a collection of items in Swift. They have some subtle differences that are worth being aware of so you can make an informed decisions when choosing between the two.
Read post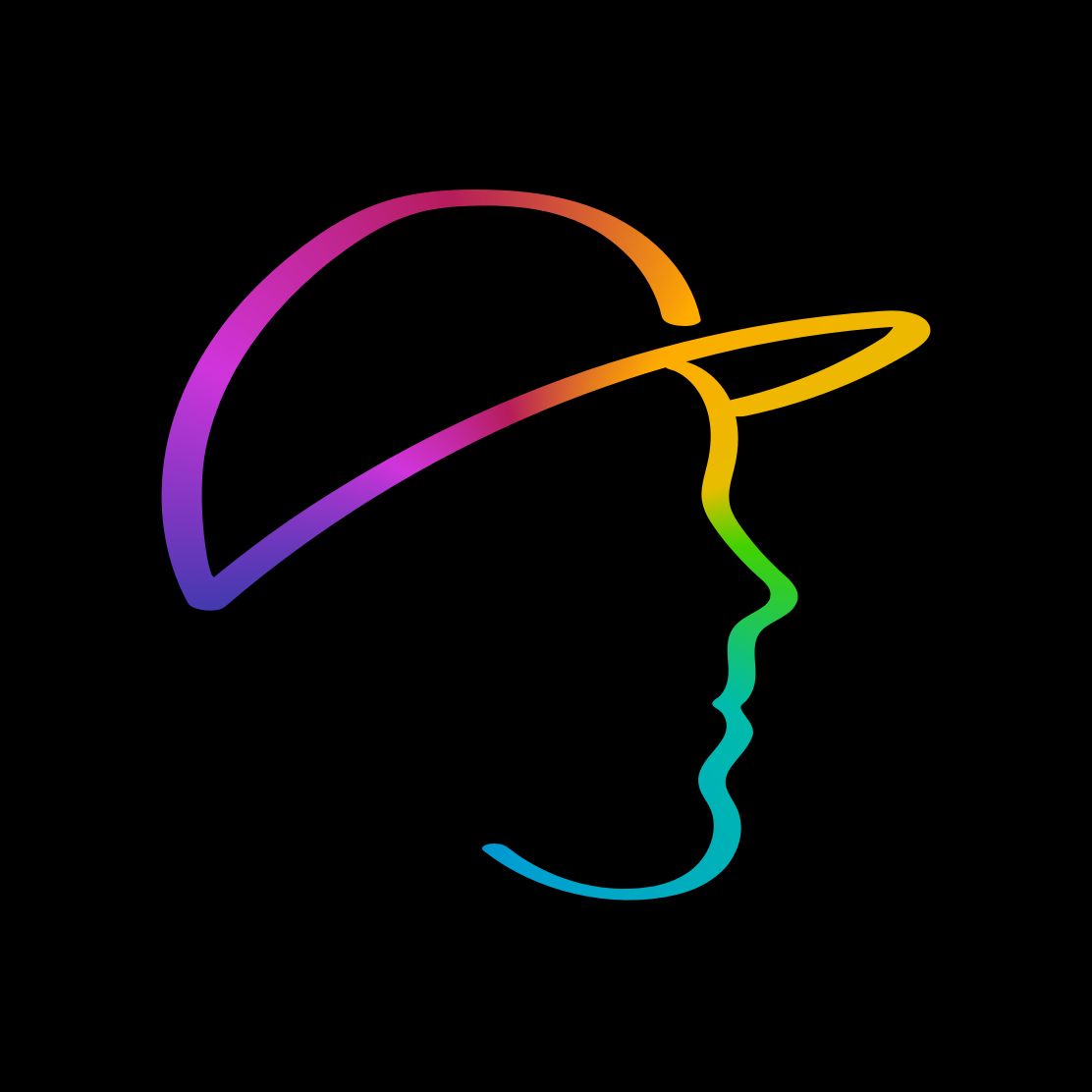
Subscribe to my newsletter and never miss a post
Learn more about Swift fundamentals
When you’re working with Arrays in Swift, it’s likely that you’ll want to sort them at some point. In Swift, there are two ways to sort an Array: Through the Comparable implementation for each element in your array By providing a closure to perform a manual/specialized comparison between elements If you have a homogenous array […]
Read postThere are several ways to initialize and configure properties in Swift. In this week’s Quick Tip, I would like to briefly highlight the possibility of using closures to initialize complex properties in your structs and classes. You will learn how you can use this approach of initializing properties, and when it’s useful. Let’s dive in […]
Read postArrays in Swift can hold on to all kinds of data. A common desire developers have when they use arrays, is to remove duplicate values from their arrays. Doing this is, unfortunately, not trivial. Objects that you store in an array are not guaranteed to be comparable. This means that it’s not always possible to […]
Read postOne of Swift’s incredibly useful features is its ability to dynamically compute the value of a property through a computed property. While this is a super handy feature, it can also be a source of confusion for newcomers to the language. A computed property can look a bit strange if you haven’t seen one before; […]
Read postThis post is a short demo of using Swift’s sort() and sort(by:) methods. For a more comprehensive overview of sorting, and some background information on how Swift’s sorting works I recommend you take a look at this updated post The easiest way to sort an Array in Swift is to use the sort method. This […]
Read postYou can use Array’s insert(_:at:) method to insert a new element at the start, or any other arbitrary position of an Array: var array = ["world"] array.insert("hello", at: 0) // array is now ["hello", "world"] Make sure that the position that you pass to the at: argument isn’t larger than the array’s current last index […]
Read postArrays in Swift are powerful and they come with many built-in capabilities. One of these capabilities is the ability to remove objects. If you want to remove a single, specific object from an Array and you know its index, you can use remove(at:) to delete that object: var array = [1, 2, 3] array.remove(at: 0) […]
Read postWhen you have an Array of elements, and you want to drop all elements that don’t match specific criteria from the Array, you’re looking for Array’s filter(isIncluded:) method. Let’s say you have an array of words and you only want to keep the words that are longer than three characters: let words = ["hello", "world", […]
Read postSwift grants developers the ability to shadow certain types with an alternative name using the typealias keyword. We can use this feature to create tuples and closures that look like types, or we can use them to provide alternative names for existing objects. In this post, we’ll look at five ways in which typealiases can […]
Read postExpand your learning with my books
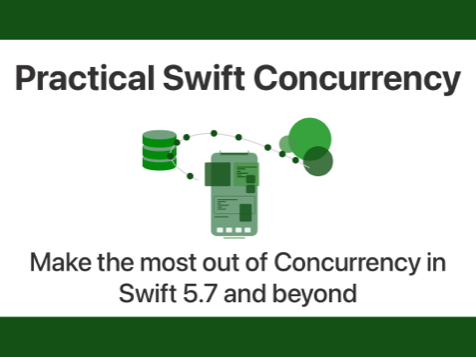
Learn everything you need to know about Swift Concurrency and how you can use it in your projects with Practical Swift Concurrency. It contains:
- Eleven chapters worth of content.
- Sample projects that use the code shown in the chapters.
- Free updates for future iOS versions.
The book is available as a digital download for just $39.99!
Learn more