When you’re decoding JSON, you’ll run into situations where you’ll have to decode dates every once in a while. Most commonly you’ll probably be dealing with dates that conform to the ISO-8601 standard but there’s also a good chance that you’ll have to deal with different date formats. In this post, we’ll take a look […]
Read post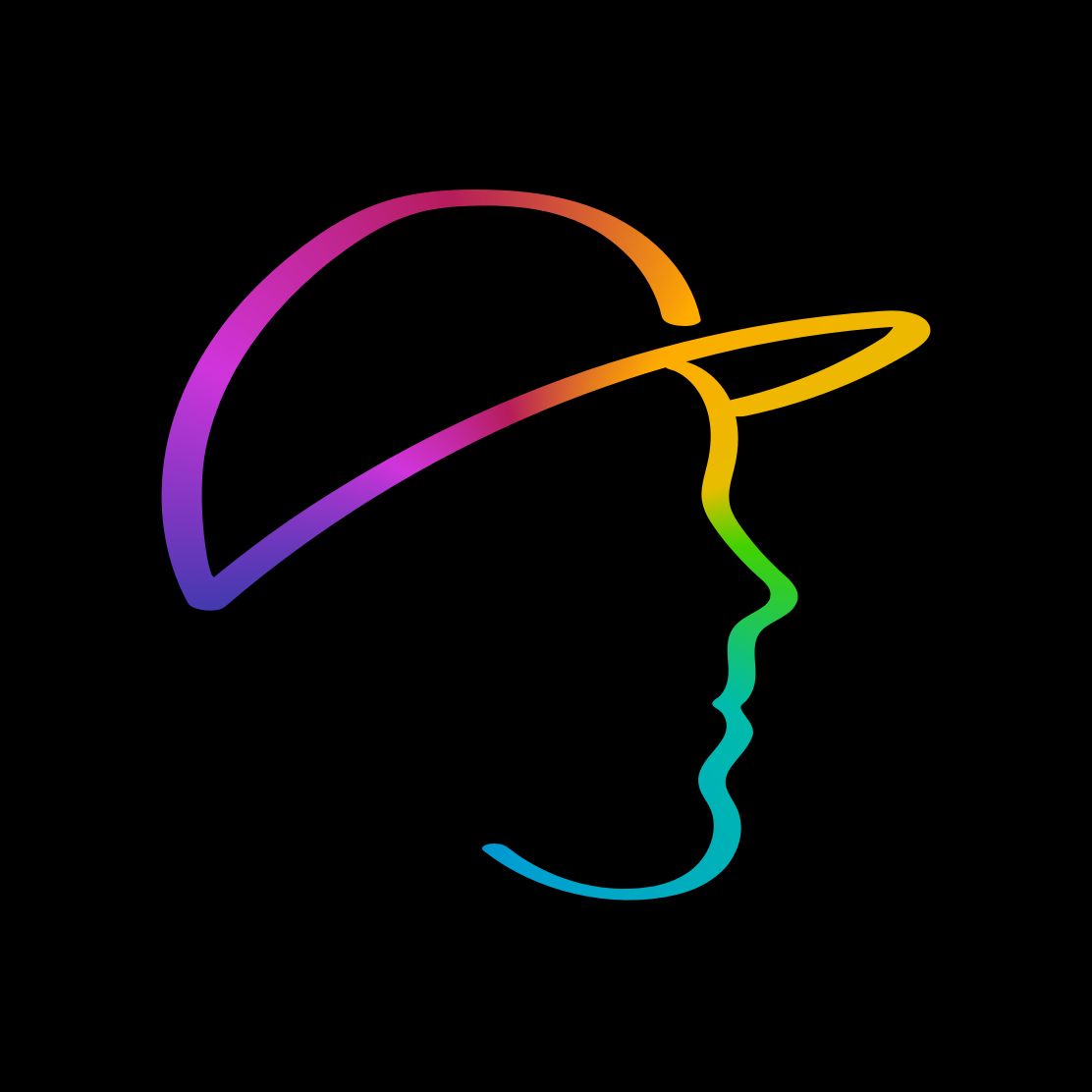
Subscribe to my newsletter and never miss a post
Learn more about Codable
Splitting a JSON object into an enum and an associated object with Codable
Published on: April 5, 2021Decoding data, like JSON, is often relatively straightforward. For a lot of use cases, you won’t need to know or understand a lot more than what I explain in this post. However, sometimes you need to dive deeper into Codable, and you end up writing custom encoding or decoding logic like I explain in this […]
Read postThe default behavior for Codable is often good enough, especially when you combine this with custom CodingKeys, it’s possible to encode and decode a wide variety of JSON data without any extra work. Unfortunately, there are a lot of situations where you’ll need to have even more control. The reasons for needing this control are […]
Read postIn the introductory post for this series you learned the basics of decoding and encoding JSON to and from your Swift structs. In that post, you learned that your JSON object is essentially a dictionary, and that the JSON’s dictionary key’s are mapped to your Swift object’s properties. When encoding, your Swift properties are used […]
Read postVirtually every modern application needs some way to retrieve, and use, data from a remote source. This data is commonly fetched by making a network request to a webserver that returns data in a JSON format. When you’re working with Javascript, this JSON data can be easily decoded into a Javascript object. Javascript doesn’t have […]
Read postOften, you’ll want you Swift models to resemble JSON that’s produced by an external source, like a server, as closely as possible. However, there are times when the JSON you receive is nested several levels deep and you might not consider this appropriate or needed for your application. Or maybe you’re only interested in a […]
Read postIf you’ve ever wanted to decode a bunch of JSON data into NSManagedObject instances you’ve probably noticed that this isn’t a straightforward exercise. With plain structs, you can conform your struct to Codable and you convert the struct from and to JSON data automatically. For an NSManagedObject subclass it’s not that easy. If your Core […]
Read postNote: After publishing this article, it has been brought to my attention that the folks from @pointfreeco have a very similar solution for the problems I outline in this post. It’s called tagged and implements the same features I cover in this post with several useful extensions. If you like this post and plan to […]
Read postYou have probably seen and used a property list file at some point in your iOS journey. I know you have because every iOS app has an Info.plist file. It’s possible to create and store your own .plist files to hold on to certain data, like user preferences that you don’t want to store in […]
Read postExpand your learning with my books
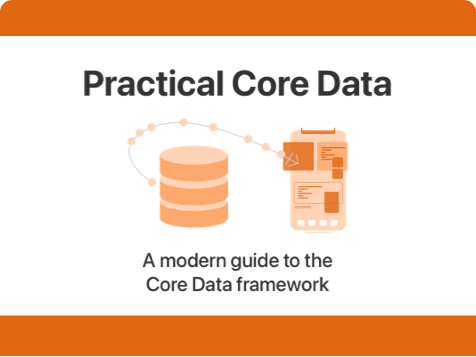
Learn everything you need to know about Core Data and how you can use it in your projects with Practical Core Data. It contains:
- Twelve chapters worth of content.
- Sample projects for both SwiftUI and UIKit.
- Free updates for future iOS versions.
The book is available as a digital download for just $39.99!
Learn more