For and forEach are both ways to loop over a collection of items in Swift. They have some subtle differences that are worth being aware of so you can make an informed decisions when choosing between the two.
Read post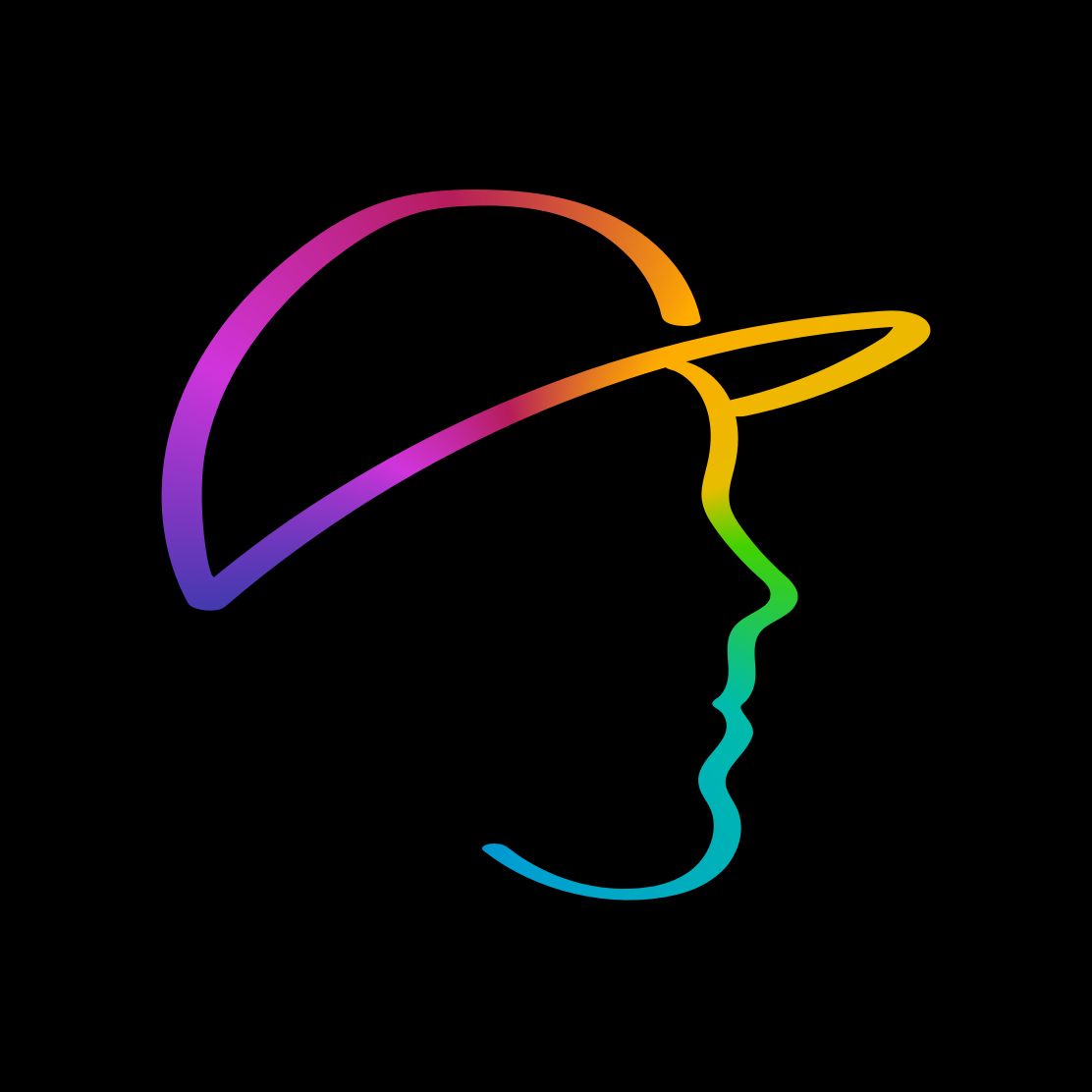
Subscribe to my newsletter and never miss a post
Learn more about Swift
Dispatching to the Main thread with MainActor in Swift
Published on: April 23, 2024Swift 5.5 introduced loads of new concurrency related features. One of these features is the MainActor annotation that we can apply to classes, functions, and properties. In this post you’ll learn several techniques that you can use to dispatch your code to the main thread from within Swift Concurrency’s tasks or by applying the main […]
Read postIf you’re keen on reading about what’s new in Swift or learn about all the cool things that are coming up, you’re probably following several folks in the iOS community that keep track and tell you about all the new things. But what if you read about an upcoming Swift feature that you’d like to […]
Read postIt’s common for developers to leverage protocols as a means to model and abstract dependencies. Usually this works perfectly well and there’s really no reason to try and pretend that there’s any issue with this approach that warrants an immediate switch to something else. However, protocols are not the only way that we can model […]
Read postThe long awaited iOS 17.4 and iPadOS 17.4 have just been released which means that we could slowly but surely start seeing alternative app stores to appear if you’re an EU iOS user. Alongside the 17.4 releases Apple has made Xcode 15.3 and Swift 5.10 available. There’s not a huge number of proposals included in […]
Read postWhen Swift 2.0 added the throws keyword to the language, folks were somewhat divided on its usefulness. Some people preferred designing their APIs with an (at the time) unofficial implementation of the Result type because that worked with both regular and callback based functions. However, the language feature got adopted and a new complaint came […]
Read postSwift’s current concurrency model leverages tasks to encapsulate the asynchronous work that you’d like to perform. I wrote about the different kinds of tasks we have in Swift in the past. You can take a look at that post here. In this post, I’d like to explore the rules that Swift applies when it determines […]
Read postWith iOS 17, we’ve gained a new way to provide observable data to our SwiftUI views. Until iOS 17, we’d use either an ObservableObject with @StateObject, @ObservedObject, or @EnvironmentObject whenever we had a reference type that we wanted to observe in one of our SwiftUI views. For lots of apps this worked absolutely fine, but […]
Read postAs we work on projects, we usually add more code than we remove. At least that’s how things are at the beginning of our project. While our project grows, the needs of the codebase change, and we start refactoring things. One thing that’s often quite hard to get exactly right when coding is the kinds […]
Read postWith iOS 17, macOS Sonoma and the other OSses from this year’s generation, Apple has made a couple of changes to how we work with data in SwiftUI. Mainly, Apple has introduced a Combine-free version of @ObservableObject and @StateObject which takes the shape of the @Observable macro which is part of a new package called […]
Read postExpand your learning with my books
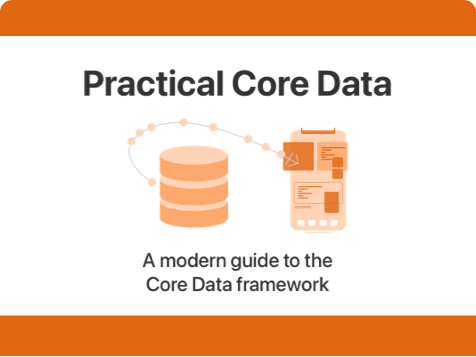
Learn everything you need to know about Core Data and how you can use it in your projects with Practical Core Data. It contains:
- Twelve chapters worth of content.
- Sample projects for both SwiftUI and UIKit.
- Free updates for future iOS versions.
The book is available as a digital download for just $39.99!
Learn more