Swift’s type system is (mostly) fantastic. Its tight constraints and flexible generics allow developers to express complicated concepts in an extremely safe manner because the Swift compiler will detect and flag any inconsistencies within the types in your program. While this is great most of the time, there are times where Swift’s strict typing gets […]
Read post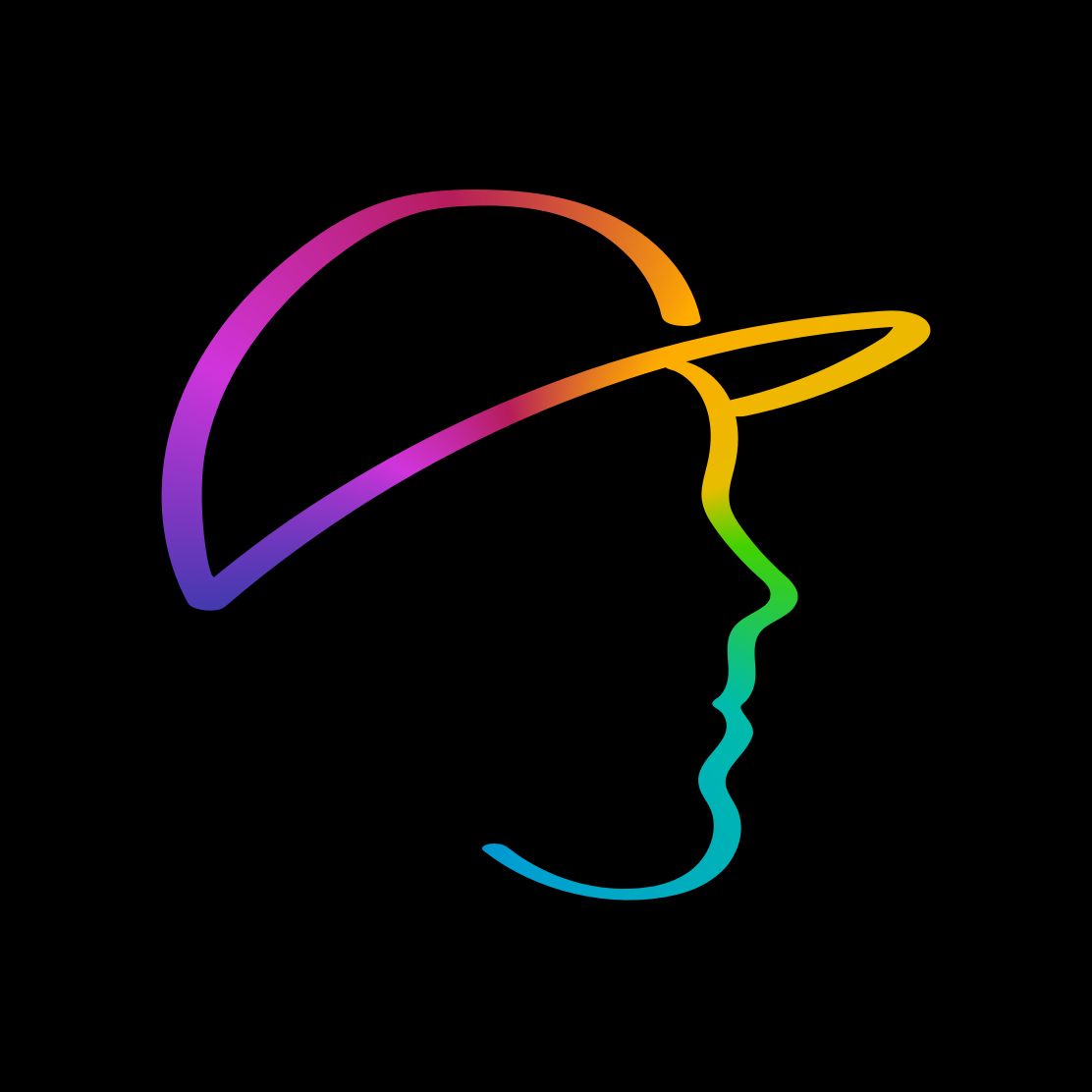
Subscribe to my newsletter and never miss a post
Learn more about Swift
Note: After publishing this article, it has been brought to my attention that the folks from @pointfreeco have a very similar solution for the problems I outline in this post. It’s called tagged and implements the same features I cover in this post with several useful extensions. If you like this post and plan to […]
Read postWhy your @Atomic property wrapper doesn’t work for collection types
Published on: April 20, 2020A while ago I implemented my first property wrapper in a code base I work on. I implemented an @Atomic property wrapper to make access to certain properties thread-safe by synchronizing read and write access to these properties using a dispatch queue. There are a ton of examples on the web that explain these property […]
Read postAn introduction to Big O in Swift
Published on: April 13, 2020Big O notation. It’s a topic that a lot of us have heard about, but most of us don’t intuitively know or understand what it is. If you’re reading this, you’re probably a Swift developer. You might even be a pretty good developer already, or maybe you’re just starting out and Big O was one […]
Read postEnforcing code consistency with SwiftLint
Published on: March 30, 2020If you’re ever amongst a group of developers and want to spark some intense discussion, all you need to do is call out that tabs are better than spaces. Or that indenting code with two spaces is much better than four. Or that the curly bracket after a function definition goes on the next line […]
Read postArrays in Swift can hold on to all kinds of data. A common desire developers have when they use arrays, is to remove duplicate values from their arrays. Doing this is, unfortunately, not trivial. Objects that you store in an array are not guaranteed to be comparable. This means that it’s not always possible to […]
Read postIf you’ve ever written or used a function that accepts a closure as one of its arguments, it’s likely that you’ve encountered the @escaping keyword. When a closure is marked as escaping in Swift, it means that the closure will outlive, or leave the scope that you’ve passed it to. Let’s look at an example […]
Read postOne of Swift’s incredibly useful features is its ability to dynamically compute the value of a property through a computed property. While this is a super handy feature, it can also be a source of confusion for newcomers to the language. A computed property can look a bit strange if you haven’t seen one before; […]
Read postUsing Result in Swift 5
Published on: March 2, 2020As soon as Swift was introduced, people were adding their own extensions and patterns to the language. One of the more common patterns was the usage of a Result object. This object took on a shape similar to Swift’s Optional, and it was used to express a return type that could either be a success […]
Read postHandling errors in Swift is done using a mechanism where functions specify their ability to throw errors. In modern Swift versions (6.0 and up), functions can even specify the type of the error that the function might throw. When we call a function that can throw, we use special syntax to either ignore the error […]
Read postExpand your learning with my books
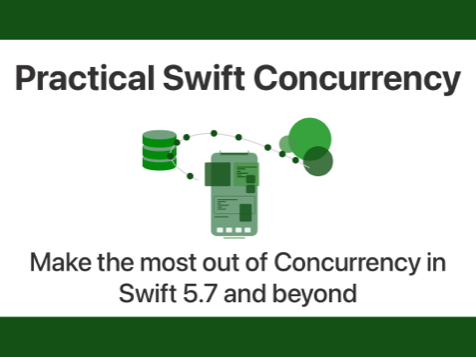
Learn everything you need to know about Swift Concurrency and how you can use it in your projects with Practical Swift Concurrency. It contains:
- Eleven chapters worth of content.
- Sample projects that use the code shown in the chapters.
- Free updates for future iOS versions.
The book is available as a digital download for just $39.99!
Learn more